函数
¥Functions
./src/index.js
文件(或 基于 TypeScript 项目中的 ./src/index.ts
文件)包括全局 register、bootstrap 和 destroy 函数,可用于添加动态和基于逻辑的配置。
¥The ./src/index.js
file (or ./src/index.ts
file in a TypeScript-based project) includes global register, bootstrap and destroy functions that can be used to add dynamic and logic-based configurations.
这些函数可以是同步的、异步的或返回一个 promise。
¥The functions can be synchronous, asynchronous, or return a promise.
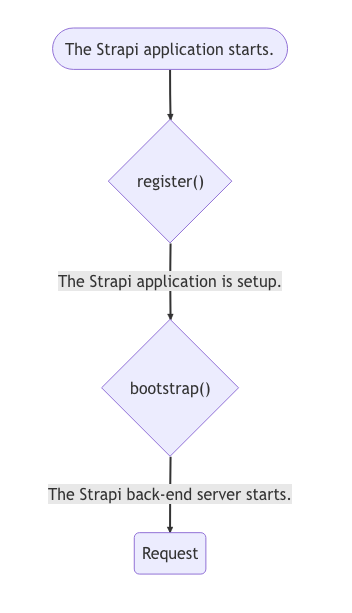
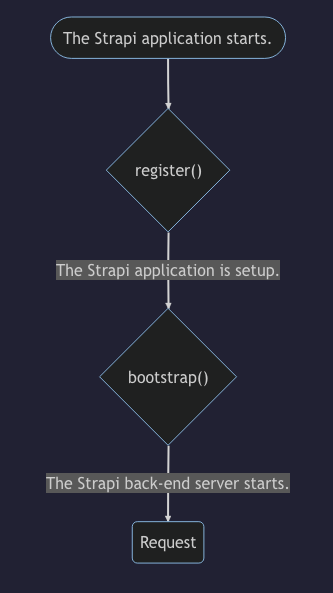
同步函数
¥Synchronous function
- JavaScript
- TypeScript
module.exports = {
register() {
// some sync code
},
bootstrap() {
// some sync code
},
destroy() {
// some sync code
}
};
export default {
register() {
// some sync code
},
bootstrap() {
// some sync code
},
destroy() {
// some sync code
}
};
异步函数
¥Asynchronous function
- JavaScript
- TypeScript
module.exports = {
async register() {
// some async code
},
async bootstrap() {
// some async code
},
async destroy() {
// some async code
}
};
export default {
async register() {
// some async code
},
async bootstrap() {
// some async code
},
async destroy() {
// some async code
}
};
返回 promise 的函数
¥Function returning a promise
- JavaScript
- TypeScript
module.exports = {
register() {
return new Promise(/* some code */);
},
bootstrap() {
return new Promise(/* some code */);
},
destroy() {
return new Promise(/* some code */);
}
};
export default {
register() {
return new Promise(/* some code */);
},
bootstrap() {
return new Promise(/* some code */);
},
destroy() {
return new Promise(/* some code */);
}
};
注册
¥Register
register
生命周期函数可在 ./src/index.js
(或 ./src/index.ts
)中找到,是一个在应用初始化之前运行的异步函数。它可用于:
¥The register
lifecycle function, found in ./src/index.js
(or in ./src/index.ts
), is an asynchronous function that runs before the application is initialized.
It can be used to:
-
以编程方式扩展 content-types
¥extend content-types programmatically
-
加载一些 环境变量
¥load some environment variables
-
注册仅由当前 Strapi 应用使用的 自定义字段,
¥register a custom field that would be used only by the current Strapi application,
-
注册 用户和权限插件的自定义提供程序。
¥register a custom provider for the Users & Permissions plugin.
register()
是 Strapi 应用启动时发生的第一件事。这发生在任何设置过程之前,并且你无权访问 register()
函数中的数据库、路由、策略或任何其他后端服务器元素。
¥register()
is the very first thing that happens when a Strapi application is starting. This happens before any setup process and you don't have any access to database, routes, policies, or any other backend server elements within the register()
function.
引导程序
¥Bootstrap
在 ./src/index.js
(或 ./src/index.ts
)中找到的 bootstrap
生命周期函数在每次服务器启动时都会被调用。
¥The bootstrap
lifecycle function, found in ./src/index.js
(or in ./src/index.ts
), is called at every server start.
它可用于:
¥It can be used to:
-
如果没有管理员用户,请创建一个
¥create an admin user if there isn't one
-
用一些必要的数据填充数据库
¥fill the database with some necessary data
-
声明 基于角色的访问控制 (RBAC) 功能的自定义条件
¥declare custom conditions for the Role-Based Access Control (RBAC) feature
bootstrapi()
函数在后端服务器启动之前、Strapi 应用设置之后运行,因此你可以访问 strapi
对象中的任何内容。
¥The bootstrapi()
function is run before the back-end server starts but after the Strapi application has setup, so you have access to anything from the strapi
object.
你可以在终端中运行 yarn strapi console
(或 npm run strapi console
)并与 strapi
对象进行交互。
¥You can run yarn strapi console
(or npm run strapi console
) in the terminal and interact with the strapi
object.
销毁
¥Destroy
destroy
函数位于 ./src/index.js
(或 ./src/index.ts
)中,是一个异步函数,在应用关闭之前运行。
¥The destroy
function, found in ./src/index.js
(or in ./src/index.ts
), is an asynchronous function that runs before the application gets shut down.
它可以优雅地用于:
¥It can be used to gracefully:
-
停止正在运行的 services
¥stop services that are running
-
清理插件操作(例如关闭连接、删除监听器等)
¥clean up plugin actions (e.g. close connections, remove listeners, etc.)