路由
¥Routes
通过任何 URL 发送到 Strapi 的请求均由路由处理。默认情况下,Strapi 为所有内容类型生成路由(参见 REST API 文档)。路由可以是 added 并配置:
¥Requests sent to Strapi on any URL are handled by routes. By default, Strapi generates routes for all the content-types (see REST API documentation). Routes can be added and configured:
-
使用 policies,这是一种阻止访问路由的方法,
¥with policies, which are a way to block access to a route,
-
以及 middlewares,这是一种控制和更改请求流和请求本身的方法。
¥and with middlewares, which are a way to control and change the request flow and the request itself.
一旦存在一条路由,到达该路由就会执行一些由控制器处理的代码(参见 控制器文档)。要查看所有现有路线及其层次顺序,你可以运行 yarn strapi routes:list
(参见 CLI 参考)。
¥Once a route exists, reaching it executes some code handled by a controller (see controllers documentation). To view all existing routes and their hierarchal order, you can run yarn strapi routes:list
(see CLI reference).
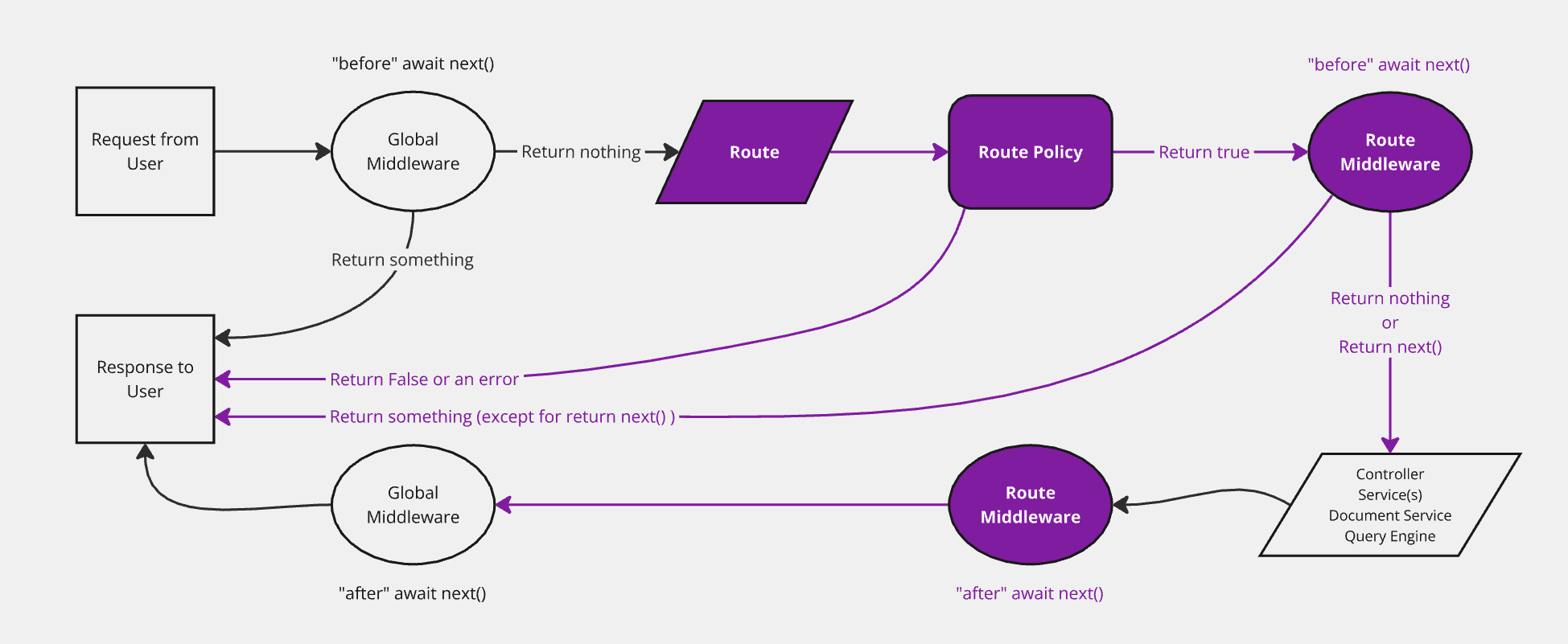
执行
¥Implementation
实现新路由包括在 ./src/api/[apiName]/routes
文件夹内的路由文件中定义它(请参阅 项目结构)。
¥Implementing a new route consists in defining it in a router file within the ./src/api/[apiName]/routes
folder (see project structure).
根据用例,有 2 种不同的路由文件结构:
¥There are 2 different router file structures, depending on the use case:
-
配置 核心路由
¥configuring core routers
-
或创建 定制路由。
¥or creating custom routers.
配置核心路由
¥Configuring core routers
核心路由(即 find
、findOne
、create
、update
和 delete
)对应于创建新 content-type 时由 Strapi 自动创建的 默认路由。
¥Core routers (i.e. find
, findOne
, create
, update
, and delete
) correspond to default routes automatically created by Strapi when a new content-type is created.
Strapi 提供了 createCoreRouter
工厂功能,可自动生成核心路由并允许:
¥Strapi provides a createCoreRouter
factory function that automatically generates the core routers and allows:
-
将配置选项传递给每个路由
¥passing in configuration options to each router
-
并禁用一些核心路由到 创建自定义的。
¥and disabling some core routers to create custom ones.
核心路由文件是一个 JavaScript 文件,使用以下参数导出对 createCoreRouter
的调用结果:
¥A core router file is a JavaScript file exporting the result of a call to createCoreRouter
with the following parameters:
范围 | 描述 | 类型 | |
---|---|---|---|
prefix | 允许传入自定义前缀以添加到该型号的所有路由(例如 /test ) | String | |
only | 只会加载的核心路由 任何不在此数组中的内容都会被忽略。 | Array | --> |
except | 不应加载的核心路由 这在功能上与 only 参数相反。 | Array | |
config | 配置处理路由的 policies、middlewares 和 公开可用性 | Object |
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
prefix: '',
only: ['find', 'findOne'],
except: [],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
},
findOne: {},
create: {},
update: {},
delete: {},
},
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
prefix: '',
only: ['find', 'findOne'],
except: [],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
},
findOne: {},
create: {},
update: {},
delete: {},
},
});
通用实现示例:
¥Generic implementation example:
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
only: ['find'],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
only: ['find'],
config: {
find: {
auth: false,
policies: [],
middlewares: [],
}
}
});
这仅允许来自核心 find
controller 的 /restaurants
路径上的 GET
请求,无需身份验证。
¥This only allows a GET
request on the /restaurants
path from the core find
controller without authentication.
创建自定义路由
¥Creating custom routers
创建自定义路由包括创建一个导出对象数组的文件,每个对象都是具有以下参数的路由:
¥Creating custom routers consists in creating a file that exports an array of objects, each object being a route with the following parameters:
范围 | 描述 | 类型 |
---|---|---|
method | 与路由相关的方法(即 GET 、POST 、PUT 、DELETE 或 PATCH ) | String |
path | 到达路径,以正斜线开头(例如 /articles ) | String |
handler | 到达路由时执行的函数。 应遵循以下语法: <controllerName>.<actionName> | String |
config 可选 | 配置处理路由的 policies、middlewares 和 公开可用性 | Object |
可以使用参数和正则表达式创建动态路由。这些参数将在 ctx.params
对象中公开。更多详细信息,请参阅 正则表达式路径 文档。
¥Dynamic routes can be created using parameters and regular expressions. These parameters will be exposed in the ctx.params
object. For more details, please refer to the PathToRegex documentation.
路由文件按字母顺序加载。要在核心路由之前加载自定义路由,请确保适当地命名自定义路由(例如 01-custom-routes.js
和 02-core-routes.js
)。
¥Routes files are loaded in alphabetical order. To load custom routes before core routes, make sure to name custom routes appropriately (e.g. 01-custom-routes.js
and 02-core-routes.js
).
Example of a custom router using URL parameters and regular expressions for routes
在以下示例中,自定义路由文件名以 01-
为前缀,以确保在核心路由之前到达该路由。
¥In the following example, the custom routes file name is prefixed with 01-
to make sure the route is reached before the core routes.
- JavaScript
- TypeScript
module.exports = {
routes: [
{ // Path defined with an URL parameter
method: 'POST',
path: '/restaurants/:id/review',
handler: 'restaurant.review',
},
{ // Path defined with a regular expression
method: 'GET',
path: '/restaurants/:category([a-z]+)', // Only match when the URL parameter is composed of lowercase letters
handler: 'restaurant.findByCategory',
}
]
}
export default {
routes: [
{ // Path defined with a URL parameter
method: 'GET',
path: '/restaurants/:category/:id',
handler: 'Restaurant.findOneByCategory',
},
{ // Path defined with a regular expression
method: 'GET',
path: '/restaurants/:region(\\d{2}|\\d{3})/:id', // Only match when the first parameter contains 2 or 3 digits.
handler: 'Restaurant.findOneByRegion',
}
]
}
配置
¥Configuration
核心路由 和 定制路由 具有相同的配置选项。路由配置在 config
对象中定义,可用于处理 policies 和 middlewares 或 公开路由。
¥Both core routers and custom routers have the same configuration options. The routes configuration is defined in a config
object that can be used to handle policies and middlewares or to make the route public.
政策
¥Policies
政策 可以添加到路由配置中:
¥Policies can be added to a route configuration:
-
通过指向
./src/policies
中注册的策略,无论是否传递自定义配置¥by pointing to a policy registered in
./src/policies
, with or without passing a custom configuration -
或者直接声明策略实现,作为一个以
policyContext
扩展 Koa 的背景 (ctx
) 和strapi
实例作为参数的函数(参见 政策文件)¥or by declaring the policy implementation directly, as a function that takes
policyContext
to extend Koa's context (ctx
) and thestrapi
instance as arguments (see policies documentation)
- Core router policy
- Custom router policy
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
policies: [
// point to a registered policy
'policy-name',
// point to a registered policy with some custom configuration
{ name: 'policy-name', config: {} },
// pass a policy implementation directly
(policyContext, config, { strapi }) => {
return true;
},
]
},
},
],
};
中间件
¥Middlewares
中间件 可以添加到路由配置中:
¥Middlewares can be added to a route configuration:
-
通过指向
./src/middlewares
中注册的中间件,无论是否传递自定义配置¥by pointing to a middleware registered in
./src/middlewares
, with or without passing a custom configuration -
或者直接将中间件实现声明为以 Koa 的背景 (
ctx
) 和strapi
实例作为参数的函数:¥or by declaring the middleware implementation directly, as a function that takes Koa's context (
ctx
) and thestrapi
instance as arguments:
- Core router middleware
- Custom router middleware
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
]
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
]
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
],
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
middlewares: [
// point to a registered middleware
'middleware-name',
// point to a registered middleware with some custom configuration
{ name: 'middleware-name', config: {} },
// pass a middleware implementation directly
(ctx, next) => {
return next();
},
],
},
},
],
};
公共路由
¥Public routes
默认情况下,路由受到 Strapi 身份验证系统的保护,该系统基于 API 令牌 或使用 用户和权限插件。
¥By default, routes are protected by Strapi's authentication system, which is based on API tokens or on the use of the Users & Permissions plugin.
在某些情况下,公开可用的路由并控制正常 Strapi 身份验证系统之外的访问可能会很有用。这可以通过将路由的 auth
配置参数设置为 false
来实现:
¥In some scenarios, it can be useful to have a route publicly available and control the access outside of the normal Strapi authentication system. This can be achieved by setting the auth
configuration parameter of a route to false
:
- Core router with a public route
- Custom router with a public route
- JavaScript
- TypeScript
const { createCoreRouter } = require('@strapi/strapi').factories;
module.exports = createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
auth: false
}
}
});
import { factories } from '@strapi/strapi';
export default factories.createCoreRouter('api::restaurant.restaurant', {
config: {
find: {
auth: false
}
}
});
- JavaScript
- TypeScript
module.exports = {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
auth: false,
},
},
],
};
export default {
routes: [
{
method: 'GET',
path: '/articles/customRoute',
handler: 'api::api-name.controllerName.functionName', // or 'plugin::plugin-name.controllerName.functionName' for a plugin-specific controller
config: {
auth: false,
},
},
],
};